JavaScript Fundementals
What are the differences between HTML and CSS
A puzzle
I see the realationship bewtween HTML and CSS as like a puzzle. HTML is like throwing the puzzle peices out of the box. The content is all there and when scattered around on a table it has all the content then. It's only when we start positioning and organizing content with CSS can we create something that functionally displays the content in a way the is palatable by the end user.
To carry on the analogy a puzzle still has all the peices if all scattered on a table. The content is all there. To me the first main difference HTML on its own can products. CSS can't position or oraganize peices without the pecies there its the same for a website.
JavaScript Flow and loop controls
Cleaning your car
The below is an example of how one might decide to clean there Car. They define how dirty the car is out of a positve number rating and then that determines how many times it needs to be cleaned. For example a car that is very dirty at a rating of 10 needs to be washed 10 times before it becomes a 0 rating of clean. The other important part of this function is that each cleaning of the car is seperate to another, once that car is cleaned the first time the dirt rating goes down by one then it decides if it needs to be cleaned again by checking if the dirt rating is greater than 0 if it is than it loops through needing to clean the car again.
What is the DOM and how to interact with it.
The DOM stand for Document Object Model. It represents the logical strucutre of a document. Can look at it this way our world is based on time.
World > Century > Decade > Year > Month > Week > Day/Date > Hour > Minute > Second
This is kinda like what the DOM does just for documents but also is a a little bit more complicated way. There are multiple faceets to the DOM and can get very complicated. The simple sort of version I like to work with can be visualized below. But again there are many more branches that span in many different directions and this is a simplified view of it.
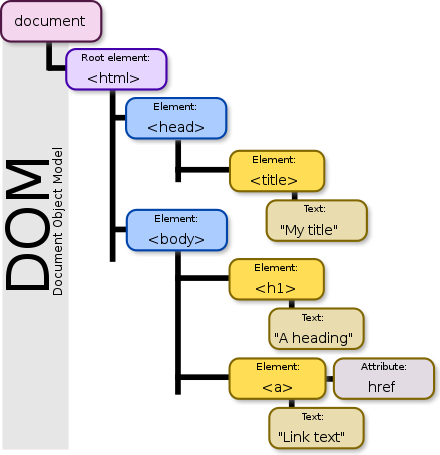
Lets interact with it
This is a JavaScript Fundementals post so the focus on interacting with it's going to be linked to JavaScript.
When interacting with the DOM in the inspection model you can right click on any element and Copy JS path what this does is copy something like the below to your clipboard. There is actually quite a few things like this in the DOM that I am picking up along the way.
document.querySelector("body > main > div:nth-child(4)"); I would not recomend doing it this way as it depends on the elements position in the document which may not always be in that position but gives you the idea of how the DOM can be useful. Something like this would be better we you can identify an ID defined in the HTML document.getElementById('blue'); This targets ID's
document.getElementsByClassName('changecolor'); This targets Classes
If you where to use this type of selector in JavaScript it would target that specfic element copied and apply any changes you wanted to it.
Essentially the DOM defines a logically process of how we can use JavaScript to target specific elemtents on a page.
Accessing Data from Arrays and Objects
An Array
An Array is a list of data. Like the below
let racketSports = ['tennis', 'squash', 'badminton', 'table tennis'] This varaible racketSports creates an array of the racket sports.
Objects
An object is a list of data that is stored against ID's. I like to view this as a simplier way to set a varaible for multiple ID's, rather than 6 varaibles for each brand of computer we can have something like the below.
let computer = {lenovo: {ram : '15gb', screen-size: '15"'}, apple: {ram : '12gb', screen-size: '14"'}}; This varaible allows us to have multiply brands of computers stored in one varaible. We can then access each brands list of data as it is a key in the data list.
How can we access them (using the variables sets above)
The way each of these are accesed are different. As you may have guessed the Object method is more complicated and is more complicated to extract the data out of.
Array list, how to get some values out of the array.
racketSports[1] the second value in the array, which is "squash"
racketSports.length the amount of values in the array
Object list, how to get some values out of the object.
computer['lenovo'] gives the whole nested values of Lenovo.
Object.keys(computer) gives all the keys that are in the list. Lenovo and Apple
What are Functions and why are the usefull
Functions are a peice of code designed to be reused, they take variables into the function and ouput a result. They are extremely usefull because they only function when called upon as well. This is a great time saver when coding. A function should be used when their is a relationship between the inputed varaible when called upon and the output. Such as how many times the car needed to be cleaned.
In my above example with washing the car that was a function. Above the function is where I called it to run. When it was called on to run I also passed through a varaible of how dirty the car was. In a completely different world someone may want to see how many times they have to clean there car if at 5 or 7 values of dirt so the function could be re-used its not staticly locked to a 10 rating of dirt.